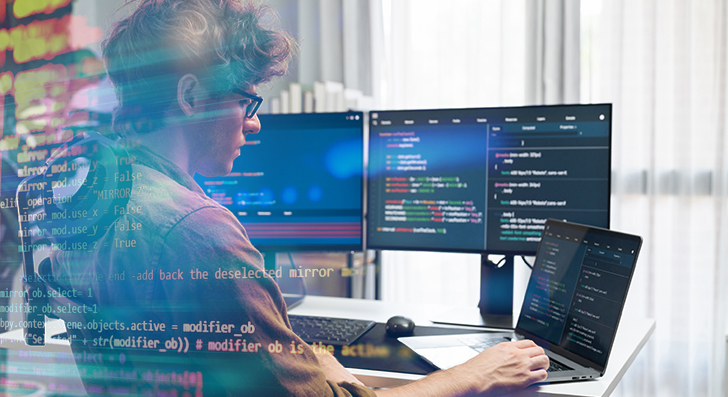
Scalability signifies your software can tackle expansion—far more customers, extra knowledge, and a lot more site visitors—without breaking. To be a developer, constructing with scalability in mind will save time and anxiety later on. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't some thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs are unsuccessful after they expand fast due to the fact the original layout can’t handle the extra load. To be a developer, you should Imagine early regarding how your system will behave under pressure.
Get started by developing your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. Alternatively, use modular structure or microservices. These patterns split your application into lesser, independent areas. Each individual module or services can scale By itself without impacting The full procedure.
Also, consider your database from day just one. Will it have to have to handle one million buyers or perhaps a hundred? Select the suitable type—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A different vital point is to prevent hardcoding assumptions. Don’t create code that only operates beneath recent conditions. Think about what would occur In case your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that support scaling, like information queues or party-pushed devices. These enable your application take care of far more requests with no receiving overloaded.
If you Make with scalability in your mind, you are not just planning for achievement—you're reducing upcoming problems. A very well-planned process is less complicated to keep up, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the appropriate Database
Choosing the right databases is usually a critical Section of creating scalable applications. Not all databases are crafted precisely the same, and using the wrong you can slow you down or perhaps cause failures as your application grows.
Begin by understanding your facts. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is an effective fit. These are definitely sturdy with relationships, transactions, and regularity. They also assist scaling methods like browse replicas, indexing, and partitioning to deal with extra targeted visitors and info.
If your knowledge is a lot more versatile—like person activity logs, product or service catalogs, or documents—take into account a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and will scale horizontally much more simply.
Also, consider your read through and generate patterns. Are you presently undertaking lots of reads with fewer writes? Use caching and browse replicas. Are you presently handling a weighty produce load? Look into databases that will cope with high compose throughput, and even celebration-centered data storage techniques like Apache Kafka (for momentary facts streams).
It’s also smart to Believe forward. You may not need to have Sophisticated scaling functions now, but picking a databases that supports them suggests you received’t have to have to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info depending on your access patterns. And always keep track of database overall performance as you develop.
In brief, the correct database depends upon your app’s construction, speed requirements, And the way you anticipate it to develop. Consider time to pick sensibly—it’ll help you save many issues afterwards.
Improve Code and Queries
Speedy code is essential to scalability. As your app grows, each and every little delay provides up. Inadequately composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Keep away from repeating logic and remove anything avoidable. Don’t select the most advanced Remedy if a simple a person performs. Keep your capabilities quick, focused, and straightforward to test. Use profiling applications to seek out bottlenecks—locations in which your code takes far too extended to operate or employs an excessive amount of memory.
Upcoming, examine your databases queries. These usually gradual items down more than the code itself. Be sure Every question only asks for the data you really have to have. Stay away from Find *, which fetches almost everything, and instead decide on unique fields. Use indexes to speed up lookups. And prevent undertaking a lot of joins, Particularly throughout significant tables.
Should you see exactly the same facts being requested time and again, use caching. Store the outcomes quickly utilizing equipment like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your databases operations whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app extra efficient.
Remember to check with massive datasets. Code and queries that do the job fine with 100 records may well crash if they have to take care of one million.
To put it briefly, scalable applications are rapidly applications. Keep the code limited, your queries lean, and use caching when desired. These steps assist your application remain easy and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of more consumers and a lot more website traffic. If all the things goes as a result of a person server, it will eventually quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support maintain your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server doing many of the do the job, the load balancer routes people to diverse servers determined by availability. This implies no single server receives overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Tools like Nginx, here HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing details briefly so it may be reused quickly. When people request the identical data once more—like an item website page or perhaps a profile—you don’t really need to fetch it with the database when. It is possible to serve it with the cache.
There are two popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) merchants data in memory for rapid access.
two. Client-facet caching (like browser caching or CDN caching) shops static data files close to the consumer.
Caching minimizes databases load, improves pace, and makes your application a lot more economical.
Use caching for things that don’t improve usually. And generally ensure your cache is current when information does transform.
In short, load balancing and caching are basic but powerful equipment. Alongside one another, they help your app cope with far more users, remain rapid, and Get better from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you require applications that let your app increase effortlessly. That’s the place cloud platforms and containers can be found in. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t should invest in components or guess upcoming capability. When site visitors will increase, you may insert additional means with just some clicks or quickly applying vehicle-scaling. When traffic drops, you can scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability applications. You could deal with building your application in lieu of running infrastructure.
Containers are A different critical Device. A container deals your app and everything it really should operate—code, libraries, options—into 1 device. This can make it effortless to move your application involving environments, from a laptop computer for the cloud, without surprises. Docker is the preferred Resource for this.
Whenever your application works by using a number of containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the app crashes, it restarts it mechanically.
Containers also help it become simple to separate aspects of your app into services. You may update or scale elements independently, which happens to be great for performance and dependability.
In brief, working with cloud and container resources usually means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you would like your application to expand without the need of limitations, get started making use of these instruments early. They save time, lessen hazard, and enable you to continue to be focused on creating, not correcting.
Monitor Almost everything
For those who don’t keep track of your application, you received’t know when things go Improper. Checking assists you see how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Section of setting up scalable systems.
Commence by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you gather and visualize this knowledge.
Don’t just watch your servers—watch your application much too. Regulate how long it takes for customers to load pages, how often mistakes occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Create alerts for crucial troubles. One example is, If the reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified quickly. This aids you resolve problems quick, often right before buyers even detect.
Monitoring is additionally helpful when you make variations. When you deploy a whole new characteristic and see a spike in glitches or slowdowns, it is possible to roll it back before it causes serious hurt.
As your app grows, traffic and facts boost. Without checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In a nutshell, checking will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it really works well, even stressed.
Final Feelings
Scalability isn’t only for huge providers. Even tiny applications want a solid foundation. By planning carefully, optimizing correctly, and utilizing the proper instruments, you are able to Make apps that expand effortlessly with out breaking stressed. Get started tiny, Assume big, and Construct clever.